POOMA ALife Data Running In An iPhone.
In the previous post we covered how to build the physics engine known as FreePOOMA on a Macintosh and how to write a simple Cocoa application that starts and stops POOMA. In this post we'll look at how to grab the data POOMA has calculated and use it in a Cocoa application.
Using C++ Code in Cocoa Objective C Programs
The first thing we need to do is enable the use of C++ code in a Cocoa program. There are two ways to do this.
The first way is to change the file extension of any Objective C file that needs to also use C++ from ".m" to ".mm". We did this in the example from the previous post.
The second way is to right click the file in the Groups & Files window and select Get Info from the popup menu. Then click the General tab. Find the section named File Type. You see a drop down list box there. Use the list box to change the file type to sourcecode.cpp.objcpp.
You only need to do one of these methods, not both.
Directly Grabbing FreePOOMA Domains
POOMA stores data in Engines and Engines have a Domain that contains the actual data. So for a given object, you want to grab its Domain in order to gain access to its data. The example below shows how to do this for a POOMA Array.
Domain<1, MyDataType>& oDomain = MyPOOMAArray.totalDomain();
As you can see, Domains are templates. The first template parameter specifies the number of dimensions in the Domain. The second template parameter specifies the data type the Domain stores. Once you have a Domain, you can pull data from it using the bracket operator, [], as shown below.
MyDataType& oMyData = oDomain[0];
From here you can perform any operation supported by the data type being used.
Parsing POOMA Data From Strings Programatically
Many POOMA objects can send their data to a stream. You can use this functionality to send POOMA data to a string stream, and then parse the resulting string. An example is shown below.
stringstream oStream;
oStream << MyPOOMAArray;
string strData = oStream.str();
parseString(strData); // custom function to parse the string and pull the needed data from it.
In the docs directory of the FreePOOMA install you will find tutorials in HTML format. Tutorial 11 discusses sending the data contained in POOMA objects to streams.
Parsing POOMA Data From Strings Manually
Finally, when debugging your program in XCode you can print POOMA data to the gdb console by sending it to the cout stream. You can then open the gdb console, copy the data and manually edit it to fit whatever format you need. Other programs can then use this data without the need to actually run POOMA. A code sample of how to do this is shown below.
cout << MyPOOMAArray; // Put a break point here.
In this case we put a breakpoint on the line of code shown above. Just before the code executes we open the gdb console and clear the log using the "Clear Log" button located at the upper right corner of the gdb console window. Then we step over the line of code shown above. Finally, we open the gdb console again and copy the resulting printout. Paste this information into TextEdit or some other editor and change it into the format you need to read it in your program.
The images below illustrate these steps. These images were taken while running a slightly modified version of the ALife example program provided with the FreePOOMA install.
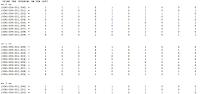
Image 1. POOMA data in the gdb console. Click image for larger picture.
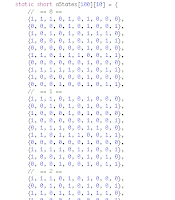
Image 2. The edited version of the gdb console data. It's now a C array. Click image for larger picture.
The movie at the beginning of this post shows the POOMA ALife data being used by an application running in an iPhone. Because we manually added the data to the program, FreePOOMA does not need to run on the iPhone.
No comments:
Post a Comment